JavaScript has come a long way since its inception, and with the release of ECMAScript 2015 (ES6), it gained a plethora of powerful features that revolutionized the way developers write code. While many of these features are well-known and widely used, some hidden gems often go unnoticed. In this blog post, we’ll take a look at these secret JavaScript ES6 features that big tech companies might not want you to know about. Whether you’re a seasoned developer or just starting your coding journey, these insights will help you write more efficient and elegant code.
The Power of Destructuring
Object Destructuring
One of the most useful features introduced in ES6 is destructuring. It allows you to extract values from objects or arrays and assign them to variables in a more concise way. Let’s take a look at object destructuring:
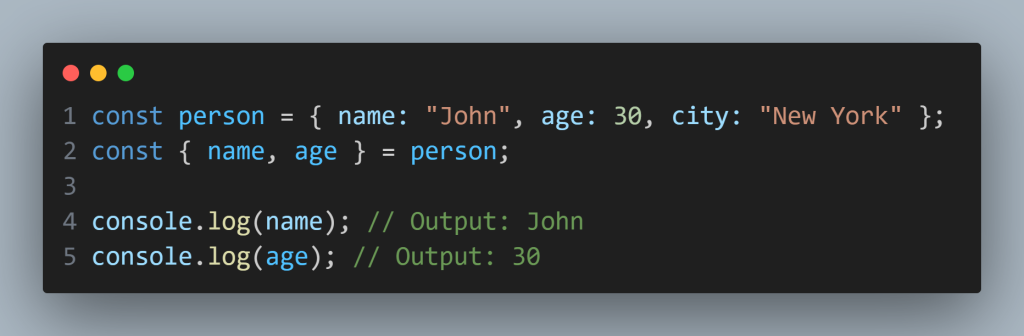
This simple technique can make your code much cleaner and easier to read, especially when working with complex objects.
Array Destructuring
Array destructuring works similarly:
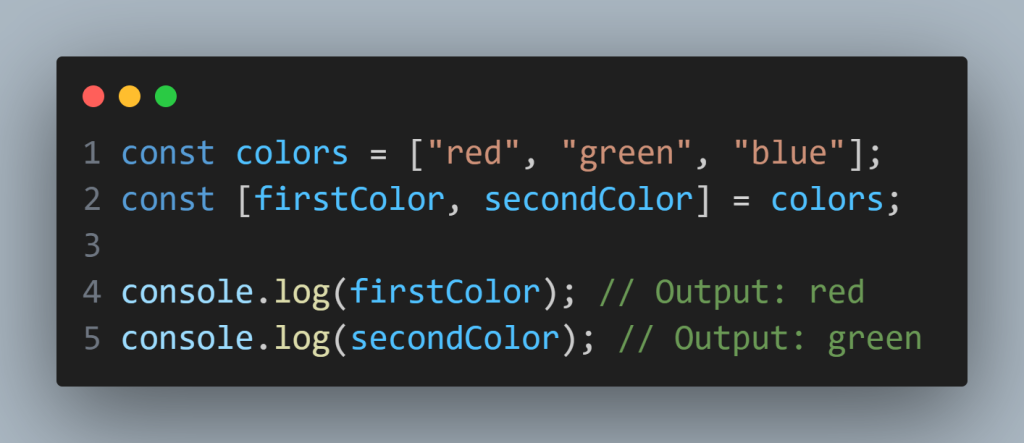
You can even use it to swap values without a temporary variable:
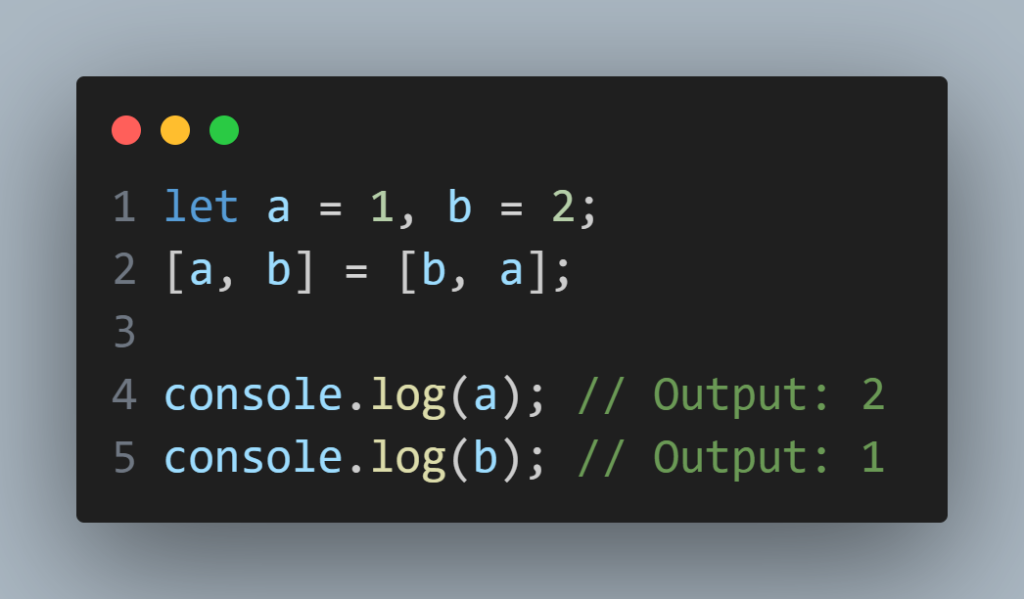
The Magic of Rest and Spread Operators
Rest Operator
The rest operator (...
) allows you to represent an indefinite number of arguments as an array. It’s incredibly useful when you want to work with a variable number of parameters:
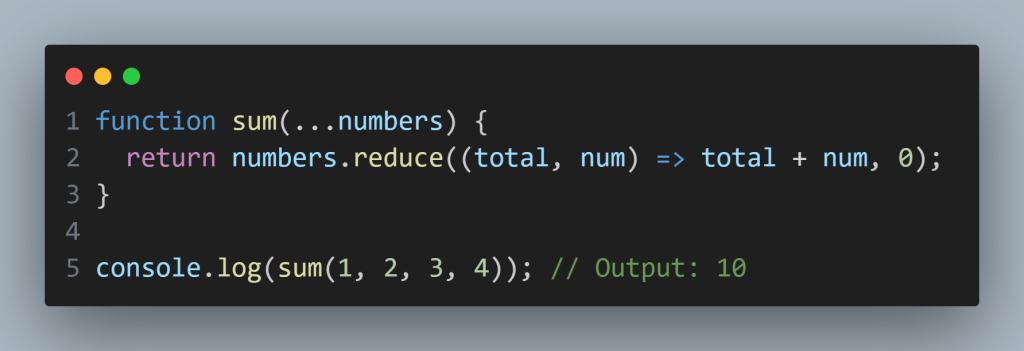
Spread Operator
The spread operator, which uses the same syntax as the rest operator, allows you to expand an iterable (like an array or string) into individual elements:
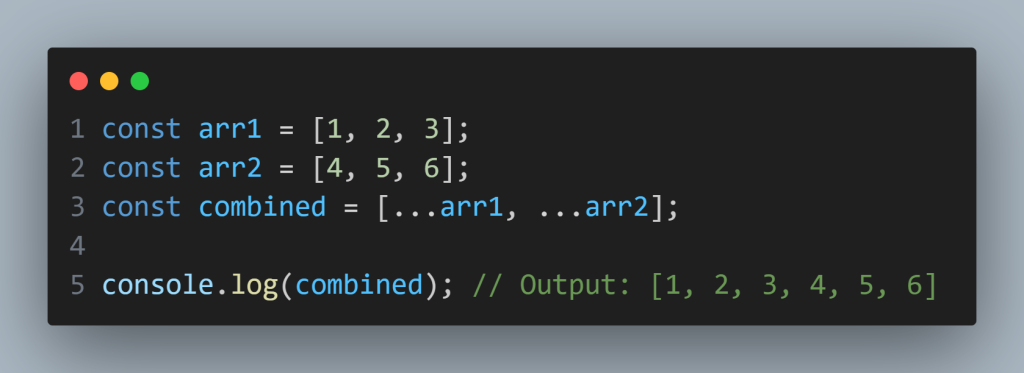
It’s also great for creating shallow copies of arrays or objects:
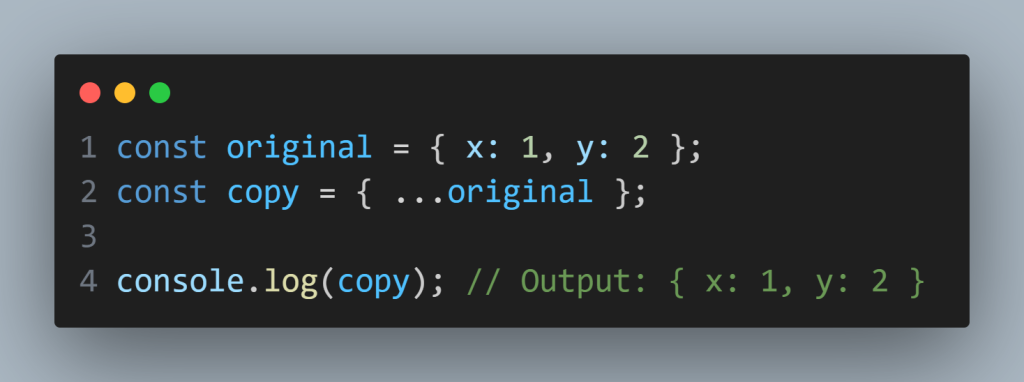
Template Literals: More Than Just String Interpolation
Template literals, enclosed in backticks (`
), are commonly employed for basic string interpolation. However, they offer a more advanced capability known as tagged templates, which enables the parsing of template literals using a function.
Tagged templates are a unique JavaScript feature that allows for custom processing of template literals. This processing is carried out by a function, typically referred to as the “tag
” which can manipulate or transform the string and its embedded expressions in a customized manner.
How Tagged Templates Work
When utilizing a tagged template, the designated “tag
” function receives the template literal as arguments. Specifically:
- The first argument is an array of the literal parts of the string (the parts that are not inside
${}
). - The remaining arguments are the values of the expressions inside
${}
.
Example of Tagged Templates
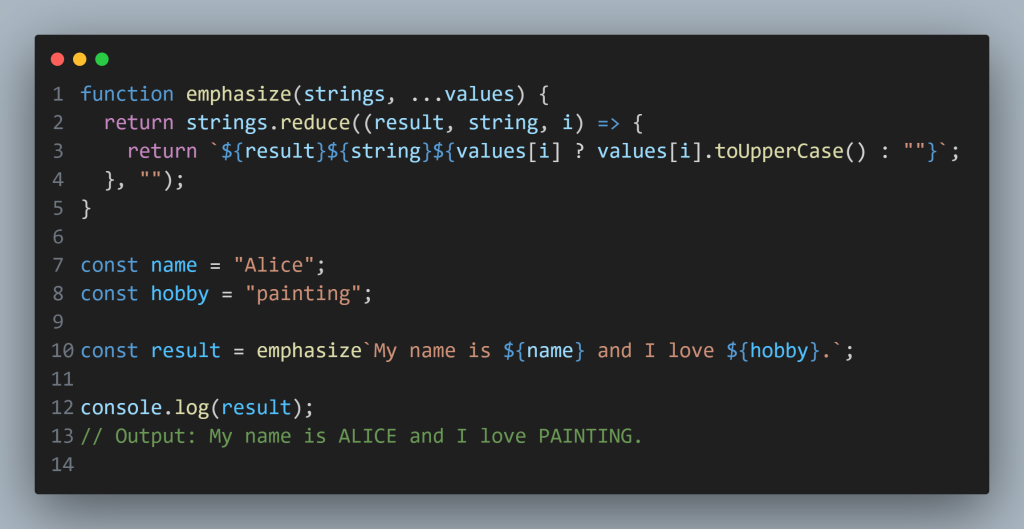
The emphasize
function serves as a tag function, processing the literal components of the template and its values. It combines these elements, transforming the values to uppercase.
The template literal "My name is ${name} and I love ${hobby}."
is handled by the emphasize
function. In the resulting output, the tag function converts the values (“Alice” and “painting”) to uppercase before integrating them back into the string.
Tagged templates offer several advantages such as:
- Dynamic string processing: They allow for custom formatting, escaping, or validation logic.
- Internationalization (i18n): They can assist with translation or string formatting based on locale.
- Input sanitization: They help prevent XSS attacks by cleaning user input before embedding it in HTML.
The Power of Default Parameters
Default parameters allow you to specify default values for function arguments:
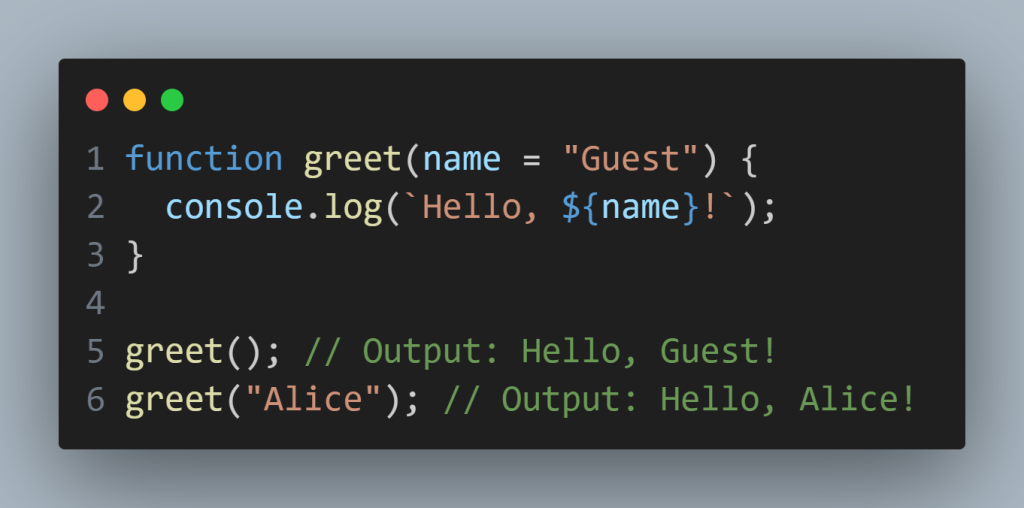
What’s less known is that you can use expressions or even function calls as default values:
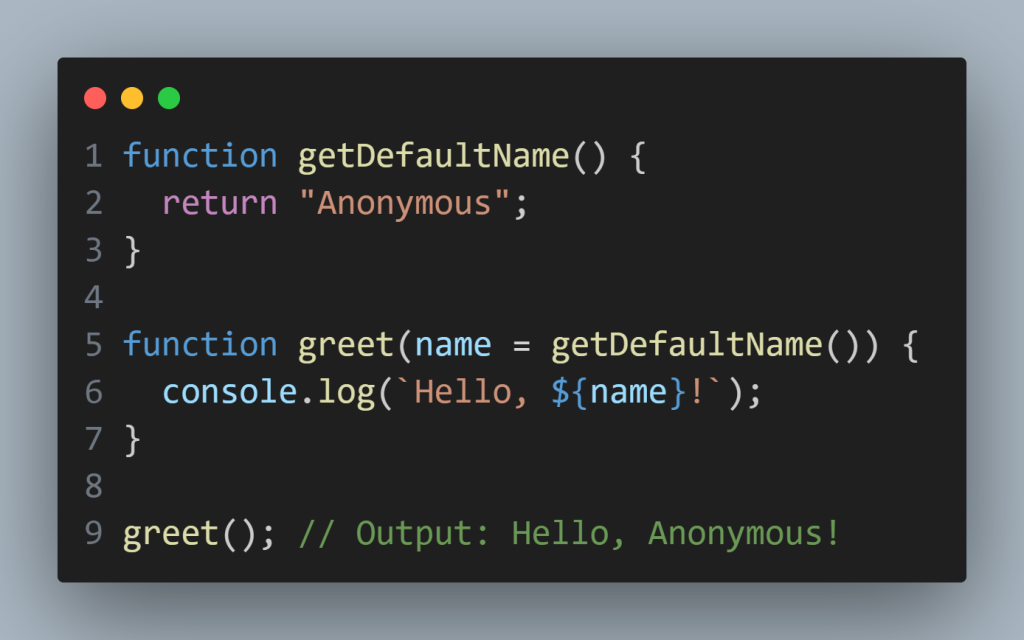
This feature allows for more dynamic and flexible function definitions.
The Versatility of Set and Map
ES6 introduced two new data structures: Set and Map. While they might seem simple at first, they have some powerful features that are often overlooked.
Set
A Set is a collection of unique values. It’s great for removing duplicates from an array:
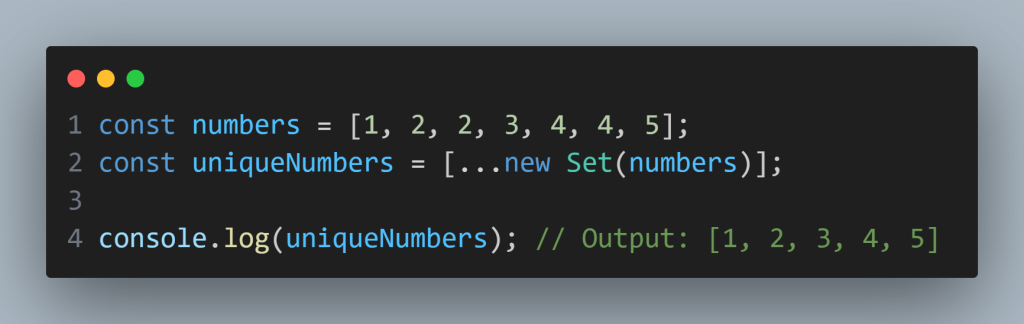
You can also use Set for efficient membership testing:
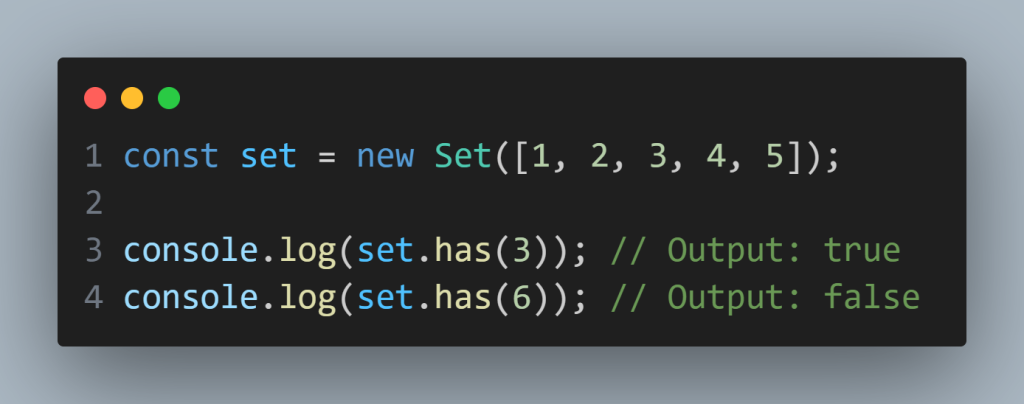
Map
Map is a collection of key-value pairs where both the keys and values can be of any type:
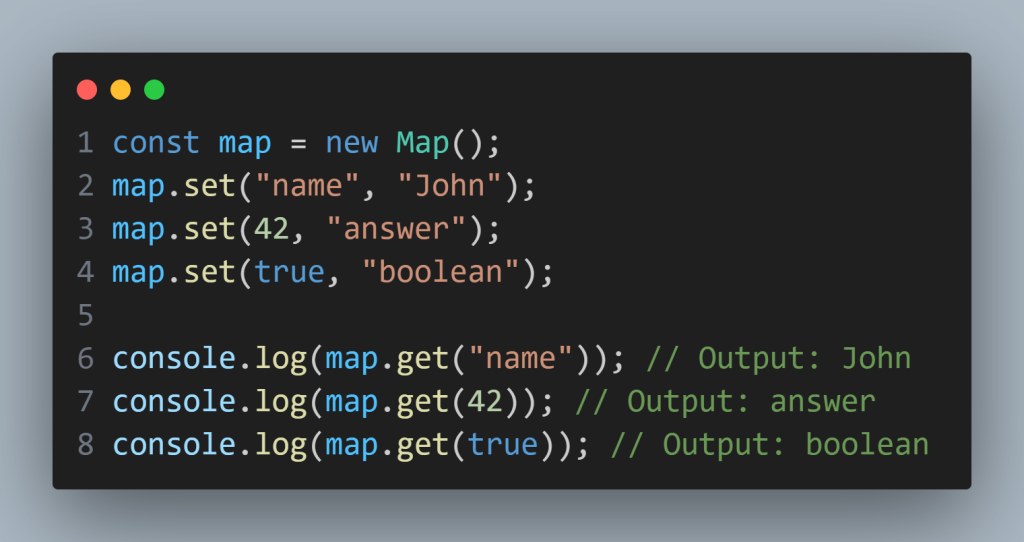
One lesser-known feature of Map is that you can use objects as keys:
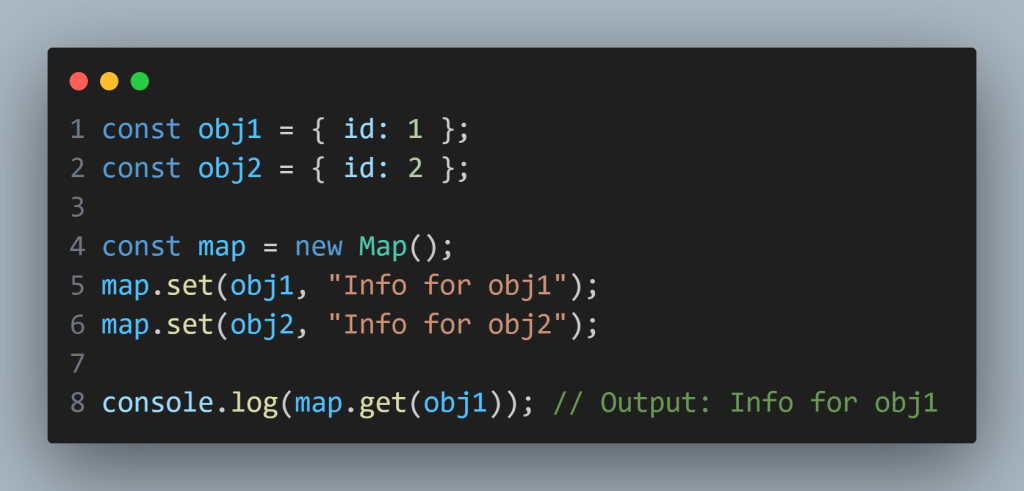
This can be incredibly useful for caching or storing metadata about objects.
The Power of Symbol
Symbols are a new primitive type introduced in ES6. They are unique and immutable, making them perfect for creating non-string property keys that won’t collide with other properties:
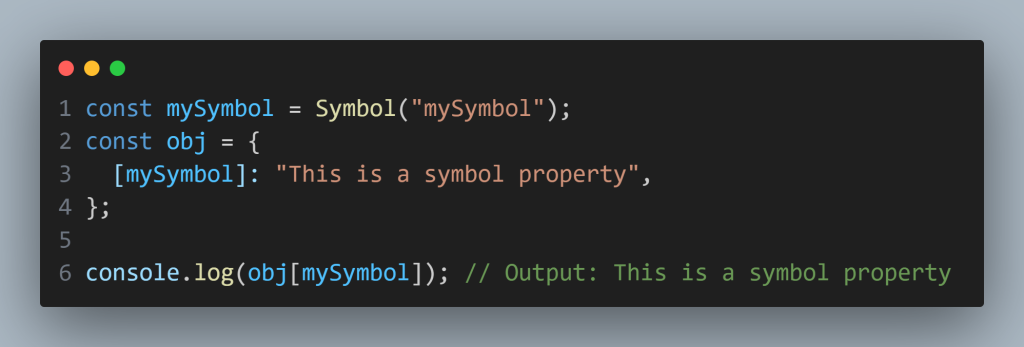
Symbols are often used for creating “hidden” properties that won’t show up in normal enumeration:
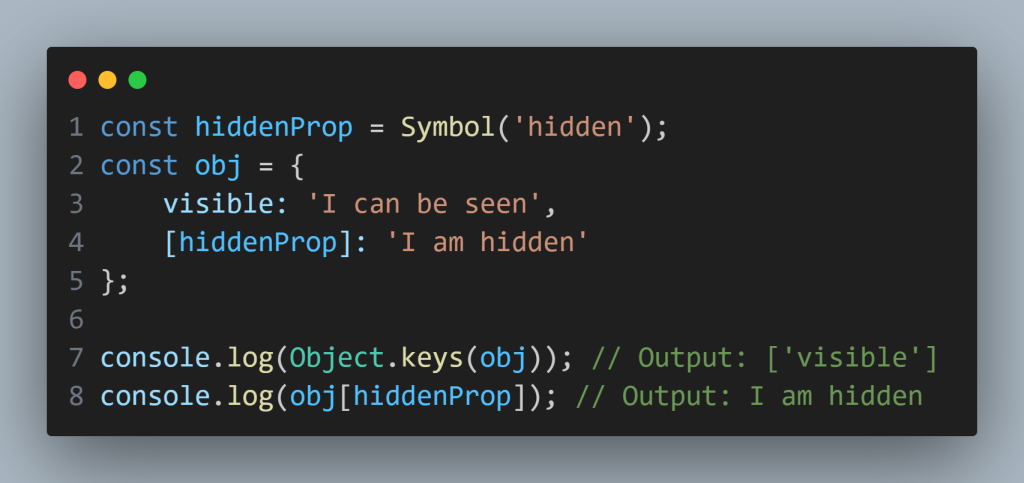
This feature can be useful for adding metadata to objects without interfering with existing properties or for implementing certain design patterns.
Conclusion
These secret JavaScript ES6 features are just the tip of the iceberg when it comes to the power and flexibility of modern JavaScript. By understanding and utilizing these lesser-known features, you can write more efficient, cleaner, and more maintainable code. Whether you’re building complex web applications or simple scripts, these tools can give you an edge in your development process.
Remember, the key to mastering JavaScript is continuous learning and practice. Don’t be afraid to experiment with these features in your projects. You might be surprised at how they can simplify your code and solve problems in elegant ways.
As you continue your JavaScript journey, keep exploring and discovering new techniques. The language is constantly evolving, and staying up-to-date with its features can help you become a more effective and innovative developer.